Functions
Overview
Glassix Functions is a serverless environment that allows you to run your code without the hassle of managing servers.
Functions enable you to create, test, and deploy code triggered by specific events, such as webhooks or directly from chatbot flows.
With Functions, the maintenance and scaling of your web infrastructure are handled seamlessly, adapting to the needs of your use case.
Key Features
- Glassix Functions provides secure access by default, allowing only Glassix requests to execute code.
- The platform offers autoscaling capabilities, dynamically adding capacity to meet the changing demands.
- To help you troubleshoot failures in a function, Glassix logs all requests handled by your function and automatically stores logs generated by your code (using console.log, console.error)
- Functions use Node 18 on Linux, providing a familiar environment for development.
- Glassix allows you to trigger your Functions based on system events. Read more on events here.
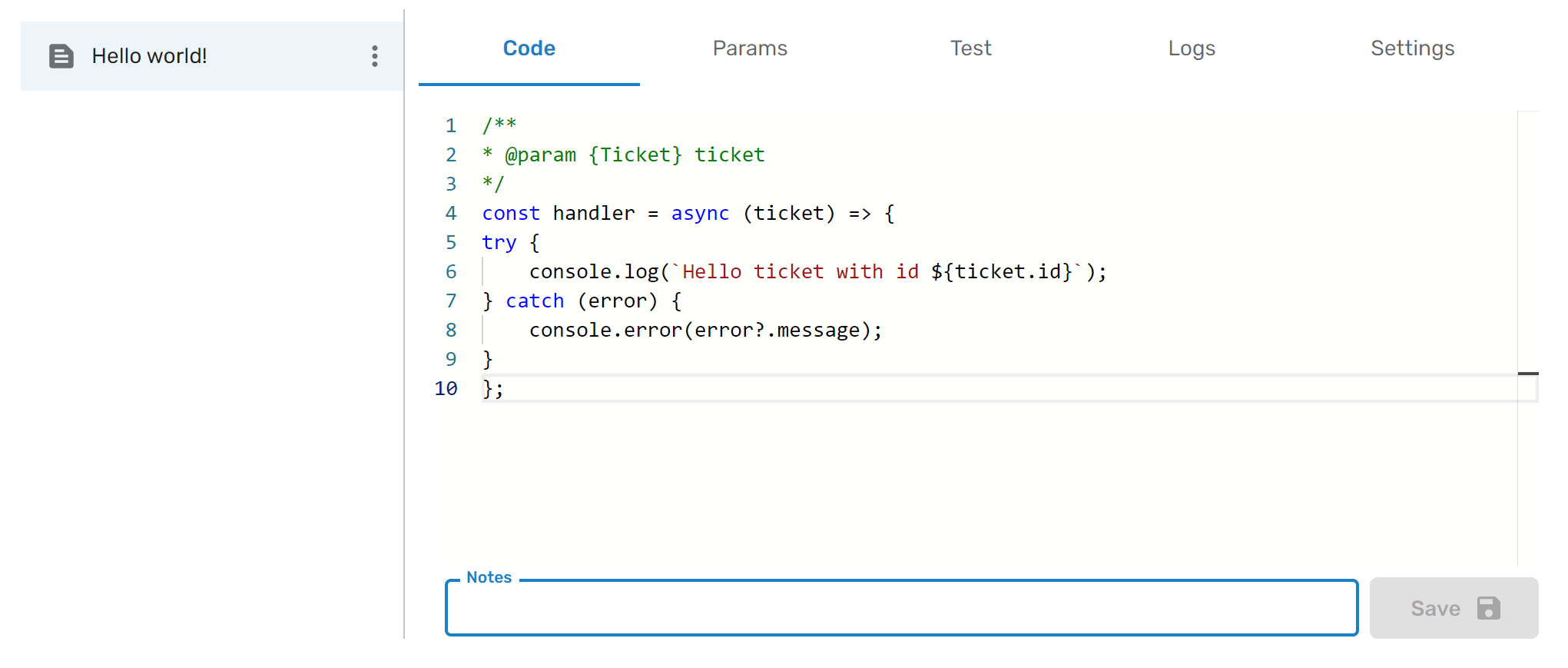
Getting Started
- Go to Settings.
Click on the symbol in the agent dashboard.
- Click on Developers → Functions. Click here
- Fill Function Name and select Category, Type, and Timeout → Click Create.
- Connect the function to a chatbot flow or a Webhook.
Info:
Learn more about connecting your functions to a chatbot here or to a Webhook here.
Code
This section of the Functions dashboard is where you input your JavaScript.
Note:
Glassix Functions supports Node.js 18 and runs on Linux. As of right now, we do not support importing modules.
Params
In this section, you can manage your function's parameters.
In parameters are the parameters your function expects to receive.
Out parameters are the parameters your function returns.
- Under In/Out parameters, click Add parameter.
- In the Name text box, type your parameter's name.
- Under Type, select the desired type.
- In the Description text box, type a description for your parameter.
- Click Save.
Test
In this section, you can test your function.
- Under In parameters in the Value text box, type your test value.
- Click Test.
Logs
In this section, you can see the logs of your function.
Info:
Be sure to wrap your function code in a try/catch statement, in order to account for unexpected errors in your code.
Examples
Validate Social Security Number
The following function checks whether an input string is a valid USA social security number. For example, "123-45-6789" is a valid social security number, and "12-34-56" is not.
It has an in-parameter named "ssn" of type String and an out-parameter named "isSsnValid" of type Boolean.
/**
* @param {Ticket} ticket
* @param {string} ssn
*/
const handler = async (ssn, ticket) => {
let isSsnValid = false;
try {
console.log(`ssn: ${ssn}`);
const ssnRegex = /^(?!000|666)[0-8][0-9]{2}-?(?!00)[0-9]{2}-?(?!0000)[0-9]{4}$/;
isSsnValid = ssnRegex.test(ssn);
console.log(`isSsnValid: ${isSsnValid}`);
} catch (error) {
console.error(error);
}
return { isSsnValid };
};
Validate URL
The following function checks whether a string is a valid URL using a regular expression.
It has an in-parameter named "url" of type String and an out-parameter named "isUrlValid" of type Boolean.
/**
* @param {Ticket} ticket
* @param {string} url
*/
const handler = async (url, ticket) => {
let isUrlValid = false;
try {
console.log(`url: ${url}`);
const urlRegex = /^[(http(s)?):\/\/(www\.)?a-z0-9@:%._\+~#=]{2,256}\.[a-z]{2,6}\b([-a-z0-9@:%_\+.~#?&//=]*)$/i;
isUrlValid = urlRegex.test(url);
console.log(`isUrlValid: ${isUrlValid}`);
} catch (error) {
console.error(error);
}
return { isUrlValid };
};
Validate License Plate Number
The following function checks whether a string is a valid USA license plate number.
It has an in-parameter named "licensePlate" of type String and an out-parameter named "isLicensePlateValid" of type Boolean.
/**
* @param {Ticket} ticket
* @param {string} licensePlate
*/
const handler = async (licensePlate, ticket) => {
let isLicensePlateValid = false;
try {
console.log(`licensePlate: ${licensePlate}`);
const licensePlateRegex = /^[A-Z0-9]{6,7}$/;
isLicensePlateValid = licensePlateRegex.test(licensePlate);
console.log(`isLicensePlateValid: ${isLicensePlateValid}`);
} catch (error) {
console.error(error);
}
return { isLicensePlateValid };
};
Reformat Date
The following function receives a string representation of a date using the format DD.MM.YYYY or DD/MM/YYYY, and reformats it to the format DD/MM/YY. For example, the input date "20.12.2023" will be reformatted to "20/12/23".
It has an in-parameter named "date" of type String and an out-parameter named "reformattedDate" of type String.
/**
* @param {Ticket} ticket
* @param {string} date
*/
const handler = async (date, ticket) => {
let reformattedDate = "";
try {
console.log(`date: ${date}`);
const dateRegex = /(\d{1,2})[\/\.](\d{1,2})[\/\.]\d*(\d{2})/;
const dateMatch = dateRegex.exec(date);
if (dateMatch) {
// Reformat the date using the matching groups of the regex
reformattedDate = `${dateMatch[1]}/${dateMatch[2]}/${dateMatch[3]}`;
console.log(`reformattedDate: ${reformattedDate}`);
} else {
console.log("Invalid date format");
}
} catch (error) {
console.error(error);
}
return { reformattedDate };
};
Check Whether a Date is in the Future
The following function checks whether a date is in the future. For example, "2040-01-01" will be considered futuristic, while "1999-01-01" will not. Keep in mind that to keep this example simple, we don't perform any validations on the input date before we pass it to the Date constructor.
The function has an in-parameter named "date" of type String and an out-parameter named "isDateFuturus" of type Boolean.
/**
* @param {Ticket} ticket
* @param {string} date
*/
const handler = async (date, ticket) => {
let isDateFuturus = false;
try {
console.log(`date: ${date}`);
// Create Date objects of the date input and of the current date
const inputDate = new Date(date);
const nowDate = new Date(Date.now());
console.log(`inputDate: ${inputDate}, nowDate: ${nowDate}`);
// The date is futurus if it is ahead of the current date
isDateFuturus = inputDate > nowDate;
console.log(`isDateFuturus: ${isDateFuturus}`);
} catch (error) {
console.error(error);
}
return { isDateFuturus };
};
Check Whether the Text is in English
The following function checks whether a text string is in English using a regular expression. In this example, a text is considered in English if it is composed only of the letters of the English alphabet and spaces.
It has an in-parameter named "freeText" of type String and an out-parameter named "isTextEnglish" of type Boolean.
/**
* @param {Ticket} ticket
* @param {string} freeText
*/
const handler = async (freeText, ticket) => {
let isTextEnglish = false;
try {
console.log(`freeText: ${freeText}`);
const isEnglishRegex = /^[a-zA-Z ]+$/;
isTextEnglish = isEnglishRegex.test(freeText);
console.log(`isTextEnglish: ${isTextEnglish}`);
} catch (error) {
console.error(error);
}
return { isTextEnglish };
};
HTTP GET Request
The following code shows how a function can send an HTTP GET request. It sends a request to the Postman Echo API along with a query parameter and logs the response data to the console.
It has an in-parameter named "queryParam" of type String.
/**
* @param {Ticket} ticket
* @param {string} queryParam
*/
const handler = async (queryParam, ticket) =>
try {
console.log(`queryParam: ${queryParam}`);
// HTTP GET request to Postman Echo API
const response = await fetch(`https://postman-echo.com/get?queryParam=${queryParam}`);
const data = await response.json();
console.log(`response: ${data.args.queryParam}`);
} catch (error) {
console.error(error);
}
};
HTTP POST Request
The following code shows how a function can send an HTTP POST request. It sends a request to the Postman Echo API with specific headers and a JSON body and logs the response data to the console.
It has an in-parameter named "bodyParam" of type String.
/**
* @param {Ticket} ticket
* @param {string} bodyParam
*/
const handler = async (bodyParam, ticket) => {
try {
console.log(`bodyParam: ${bodyParam}`);
// HTTP POST request to Postman Echo API
const response = await fetch("https://postman-echo.com/post", {
method: "POST",
headers: {
"Accept": "application/json",
"Content-Type": "application/json",
},
body: JSON.stringify({ bodyParam: bodyParam }),
});
const data = await response.json();
console.log(`response: ${data.data.bodyParam}`);
} catch (error) {
console.error(error);
}
};
Parallel HTTP Requests
The following code shows how a function can send multiple HTTP requests in parallel without having to wait for each request before sending the next one.
/**
* @param {Ticket} ticket
*/
const handler = async (ticket) => {
try {
// Parallel HTTP requests to Postman Echo API
let promise1 = fetch(`https://postman-echo.com/get?index=${1}`);
let promise2 = fetch(`https://postman-echo.com/get?index=${2}`);
let promise3 = fetch(`https://postman-echo.com/get?index=${3}`);
// Wait for all the responses
const [response1, response2, response3] = await Promise.all([promise1, promise2, promise3]);
promise1 = response1.json();
promise2 = response2.json();
promise3 = response3.json();
// Wait for the data of all the responses
const [data1, data2, data3] = await Promise.all([promise1, promise2, promise3]);
console.log(`index1: ${data1.args.index}`);
console.log(`index2: ${data2.args.index}`);
console.log(`index3: ${data3.args.index}`);
} catch (error) {
console.error(error);
}
};
Updated 2 months ago